Laser Tripwire Experiment with Phototransistor
Note: If you haven’t read about why I’m doing this, check out this post.
Goal: Build a Laser Tripwire
So the first step toward building my tennis ball sensing board was to test the simplest iteration of the sensor concept: A single laser/sensor combo, that is tripped when an object passes between them, blocking the laser beam. Will an object breaking this beam cause enough of a drop in the phototransistor’s reading to create a digital signal that we can feed into a microprocessor?
First Pass
I based my test setup on the setup in this article about making a laser tripwire with an Arduino, laser and photo-resistor. Instead of the photo-resistor, I used a phototransistor, which I set inside of a black tube (a black straw wrapped in duct tape), to protect it from ambient light. Across from this, I had my laser diode, set in a black tube, aimed directly into the transistor’s straw. Instead of the buzzer in the Instructables setup, I simply looked at the Serial Monitor in the Arduino IDE to check the readings.
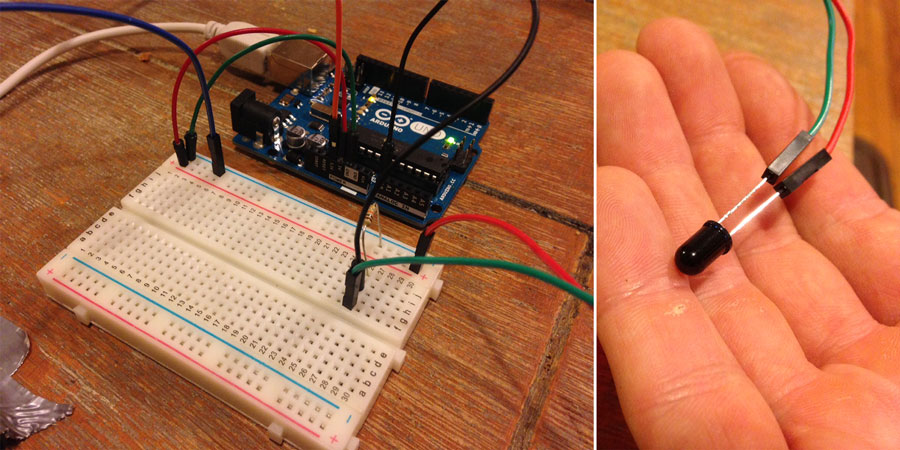
Wiring setup (Left) and a picture of the 4mA Phototransistor (Right).
Component List
- 1 Arduino Uno (connected to MacBook Pro with usb)
- 1 Breadboard
- Jumper wires
- 1 4mA Phototransistor: 512-QSD123
- 1 Laser Diode
- 1 10k Ohm Resistor
- 1 100k Ohm Resistor (2nd Pass)
10k Ohm Pulldown Resistor using analogRead() Sketch
Here’s the code for the initial test.
void setup() { pinMode(4, OUTPUT); Serial.begin(115200); } void loop(){ digitalWrite(4, HIGH); Serial.println(analogRead(0)); if (analogRead(0) < 10){ Serial.write("BEAM BLOCKED"); } }
Then I let the sketch run, and flicked my finger quickly through the beam, resulting in readings like this in the Serial Monitor:
Results
47 48 47 44 34 20 9 BEAM BLOCKED 2 BEAM BLOCKED 1 BEAM BLOCKED 0 BEAM BLOCKED 0 BEAM BLOCKED 0 BEAM BLOCKED 35 44 47 47 47 47 47
So inside the black tube setup, with the laser aimed at it, the phototransistor was transmitting readings of around 45. When I flicked my finger through, the readings went down to around 0. So it worked!
Well, kind of. At 115200 bps, my finger flicking through the beam blocked the sensor for only 6 readings. My design currently called for reading input from 72 inputs, within 1 millisecond. And the ball may be passing the sensing plane at a speed greater than my finger was moving. Although it is also larger than my finger. Still, it seems speed is going to be a concern.
I do know that analogRead() is fairly slow. Perhaps if I can use digitalRead() or digitalReadFast(), I can speed up the process. But phototransistors transmit analog signals, so I’ll have to find a way to turn that into a digital signal as well.
Problem
As it stood, my unblocked reading of ~40/1023 analog, was not enough to produce an ON reading with digitalRead(). I need the equivalent of > 625 to produce an ON reading, and < 300 to produce an OFF reading. So my ~40 unblocked dropping to 0 blocked produces an OFF reading either way.
Second Pass
Increasing Phototransistor Sensitivity
I needed a way to increase the sensitivity of a phototransistor. It turns out, one way to do this is to increase the value of your pulldown resistor, if you’re using one. It’s important to note that increasing the sensitivity of a phototransistor will make it more more sensitive to ambient light as well, which could result in false positives. However, as I’m setting my phototransistors deep into narrow black tubes, I’m not too worried about ambient light.
So I swapped out my 10k ohm resistor for a 100k ohm resistor, then tested it with analogRead() and was seeing unblocked readings in the 800s, and blocked readings still near zero. Awesome! I then rewired the circuit to feed into digital pin 2, changed the code to use digitalRead(), using the sketch below.
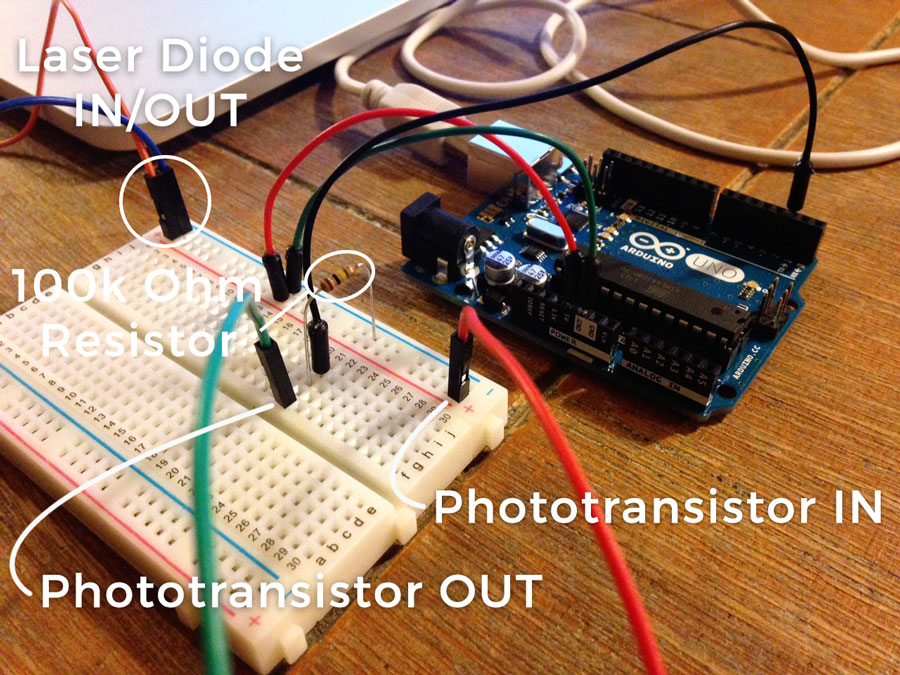
2nd attempt at the laser tripwire wiring. This time with a 100k Ohm pulldown resistor, and the phototransistor connecting to a digital input.
100k Ohm Pulldown using digitalRead() Arduino Sketch
void setup() { //5v power pinMode(4, OUTPUT); //Digital Pin pinMode(2, INPUT); //Serial monitor Serial.begin(9600); } void loop(){ digitalWrite(4, HIGH); Serial.println(digitalRead(2)); if (digitalRead(2) < 1){ Serial.write("BEAM BLOCKED"); } }
Success!
Voila! Now my unblocked beam was reading 1 at digital pin 2, and when blocked, it read a 0.
So with a successful test of a single laser/transistor tripwire, my next task will be to create an array of these, feeding into some daisy-chained 74HC165 Parallel-In Serial-Out Shift Registers and see how quickly I can read an array of these.